PCB Stands for in Assembly Language: Understanding the Meaning and Usage
PCB stands for Printed Circuit Board, which is one of the most important components of electronic devices. It is a flat board made of non-conductive material with conductive pathways etched onto its surface. The pathways connect different components on the board, such as resistors, capacitors, and integrated circuits, to form a functional circuit.
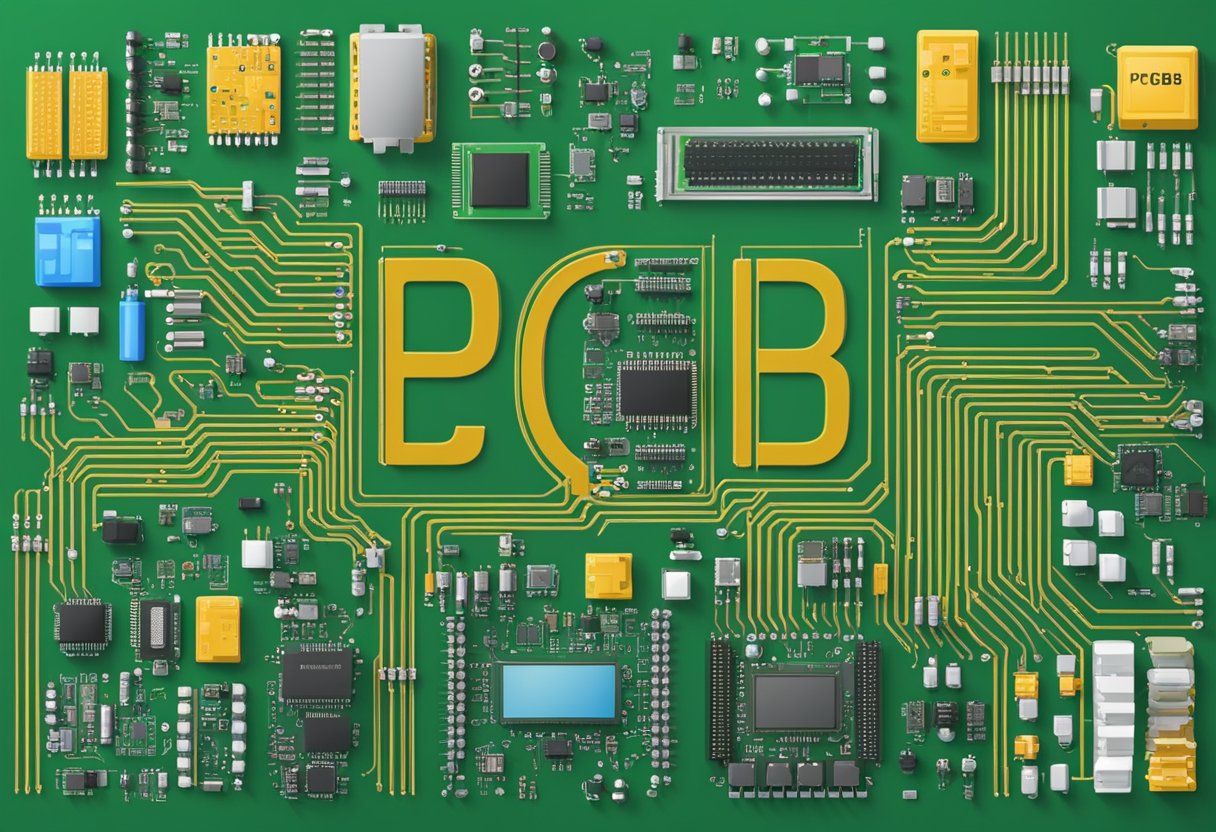
In assembly language, PCB stands for Program Counter Block. The Program Counter (PC) is a register that stores the memory address of the next instruction to be executed in a program. The PCB is a data structure that contains information about the state of a process, including the PC value, as well as other important information such as the process ID, priority, and memory allocation.
Understanding the role of PCBs in both electronic devices and assembly language programming is crucial for anyone interested in these fields. In this article, we will explore the different meanings of PCB and their significance in these contexts. We will also discuss the various types of PCBs, their design, and their applications in modern electronics.
Basics of PCB in Assembly Language
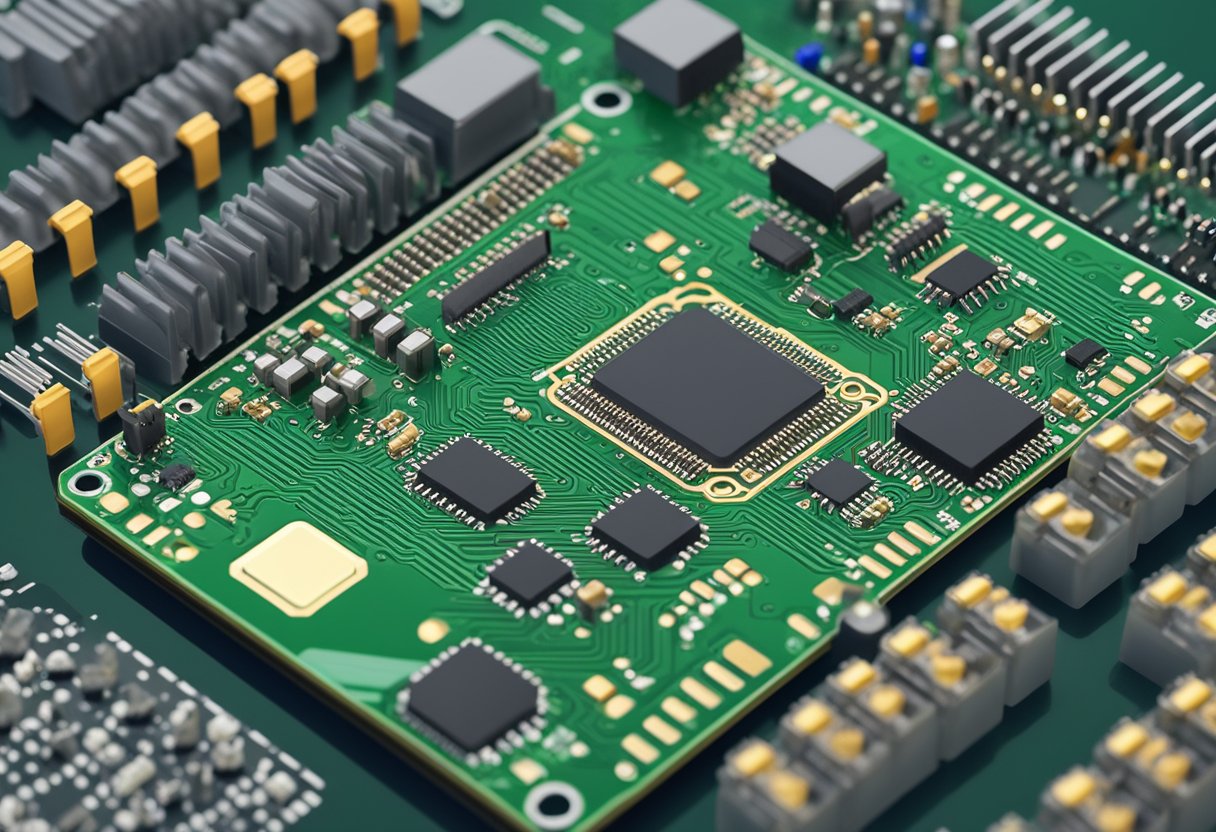
PCB stands for Printed Circuit Board. It is a board made of non-conductive material with conductive pathways etched onto it. PCBs are commonly used in electronic devices to connect different components together.
In assembly language, PCB refers to the physical layout of a circuit board and the instructions needed to assemble it. This includes the placement of components, the routing of traces, and the creation of a bill of materials.
To create a PCB assembly language, one must first design the circuit schematic. This involves creating a diagram of the circuit and the components needed to build it. Once the schematic is complete, the designer can then create a layout of the PCB, placing the components and routing the traces to connect them.
There are various software tools available for designing PCBs in assembly language, such as Altium Designer, Eagle PCB, and KiCAD. These tools allow designers to create a virtual representation of the PCB and test it before physically assembling it.
Overall, PCBs are an essential component in electronic devices and assembly language plays a crucial role in their design and creation.
PCB Definition and Components
In assembly language, PCB stands for “Program Counter Block.” A PCB is a data structure used by the operating system to store information about a process. It contains information such as the process ID, priority, state, and other details needed by the operating system to manage the process.
Register Usage
The PCB contains several registers that are used by the operating system to manage the process. One of the most important registers in the PCB is the Program Counter (PC) register. The PC register contains the memory address of the next instruction to be executed by the process.
Other registers in the PCB include the stack pointer (SP) register, which points to the top of the process’s stack, and the base pointer (BP) register, which points to the base of the process’s stack. The PCB also contains registers for storing the process’s general-purpose registers, such as the AX, BX, CX, and DX registers.
Flags and Interrupts
The PCB also contains flags and interrupt information that is used by the operating system to manage the process. The flags register contains information about the current state of the process, such as whether it is currently running, waiting, or blocked.
The PCB also contains information about the interrupts that are currently enabled for the process. Interrupts are signals that are sent to the CPU to indicate that an event has occurred that requires the CPU’s attention. By enabling and disabling interrupts, the operating system can control the flow of execution for the process.
In summary, the PCB is a critical data structure used by the operating system to manage processes in assembly language. It contains important information such as the process ID, priority, state, and other details needed by the operating system to manage the process. The PCB also contains registers for storing the process’s general-purpose registers, as well as flags and interrupt information used by the operating system to manage the process.
PCB Lifecycle in Assembly
Creation
When a process is created in assembly language, the operating system creates a Process Control Block (PCB) to keep track of the process. The PCB contains information about the process, such as its current state, priority, and memory allocation. The PCB is created and initialized by the operating system and is stored in memory.
Execution
During execution, the PCB is used by the operating system to keep track of the process’s state. The state can be running, waiting, or blocked. When a process is running, the PCB contains information about the CPU registers, program counter, and stack pointer. This information is used by the operating system to switch between processes and to save and restore the state of a process when it is interrupted.
Termination
When a process terminates, the PCB is deleted by the operating system. The memory allocated to the process is released, and any resources held by the process are freed. The termination of a process can be voluntary or involuntary. A process can voluntarily terminate by calling an exit system call, while an involuntary termination can occur due to an error or a signal from the operating system.
In summary, the PCB is a data structure used by the operating system to keep track of a process’s state during its lifecycle. It provides information about the process’s current state, priority, and memory allocation. During execution, the PCB is used to save and restore the state of a process when it is interrupted. When a process terminates, the PCB is deleted by the operating system, and the resources held by the process are freed.
Assembly Language Syntax for PCB Management
PCB stands for Printed Circuit Board, which is an essential component in electronic devices. Assembly language is a low-level programming language that is used to communicate with the computer hardware directly. In order to manage PCBs using assembly language, one must be familiar with the syntax used for PCB management.
To manage PCBs in assembly language, one must use the following syntax:
1. Defining the PCB
To define a PCB, one must use the following syntax:
PCB_name PCB
Here, PCB_name is the name of the PCB, and PCB is the keyword used to define the PCB.
2. Defining the Attributes of the PCB
To define the attributes of the PCB, one must use the following syntax:
PCB_name.attribute_name attribute_value
Here, PCB_name is the name of the PCB, attribute_name is the name of the attribute, and attribute_value is the value of the attribute.
3. Accessing the PCB Attributes
To access the attributes of the PCB, one must use the following syntax:
PCB_name.attribute_name
Here, PCB_name is the name of the PCB, and attribute_name is the name of the attribute.
4. Example
Here is an example of how to manage a PCB in assembly language:
PCB1 PCB
PCB1.priority 1
PCB1.state ready
PCB1.next PCB2
PCB2 PCB
PCB2.priority 2
PCB2.state waiting
PCB2.next PCB3
PCB3 PCB
PCB3.priority 3
PCB3.state running
PCB3.next null
In this example, three PCBs are defined, each with their own attributes. The first PCB has a priority of 1, is in the ready state, and points to the second PCB. The second PCB has a priority of 2, is in the waiting state, and points to the third PCB. The third PCB has a priority of 3, is in the running state, and has no next PCB.
By following the syntax for PCB management in assembly language, one can effectively manage PCBs in electronic devices.
Common PCB Operations in Assembly
Context Switching
Context switching is a process that allows the operating system to switch between different processes efficiently. It is a critical operation that occurs when the CPU switches from executing one process to another. During context switching, the operating system saves the context of the current process and restores the context of the next process to be executed.
The context of a process includes the values of all the CPU registers, the program counter, and the stack pointer. The operating system saves this information in the process control block (PCB) of the current process and restores it from the PCB of the next process.
Process Scheduling
Process scheduling is another essential operation that occurs in the operating system. The operating system schedules processes to run on the CPU based on their priority and other factors such as the amount of CPU time they have used and the amount of memory they require.
The PCB of a process contains information about its priority, its state (running, waiting, or blocked), and the resources it is using. The operating system uses this information to decide which process to run next.
In summary, the PCB is a vital data structure used by the operating system to manage processes efficiently. It contains information about the state of a process, the resources it is using, and its priority. Context switching and process scheduling are two critical operations that rely on the PCB to manage processes effectively.